To input languages with numerous characters or requiring character combinations, such as Chinese, Japanese, or Korean (Hangul), a special input method is needed in Linux1. In Windows or macOS, these input methods are integrated into the operating system by default, so users don’t need to worry about them. In Linux, however, depending on the distribution, users often need to install and configure input methods separately.
When inputting non-Western languages in Linux environments, issues related to input methods occasionally arise. For developers to effectively solve these problems, an understanding of Linux’s input method system is necessary.
In this article, we’ll examine in detail the roles and interactions of various components that make up Linux’s input method system, as well as how they operate.
1. Input Methods and Input Method Frameworks
Typically, input methods operate on top of an input method framework. An input method framework is higher-level software that manages and integrates various input methods, with IBus and Fcitx being the most prominent examples.
Input methods themselves generally need to be installed separately from the input method framework.
For example, to use a Fcitx-based Korean input method, you need to install the fcitx5-hangul
package, and for an IBus-based Japanese input method, the ibus-mozc
package.
The input method framework acts as an intermediary, routing key inputs from applications to the activated input method according to user settings. For convenience, in this article, we’ll refer to both input method frameworks and input methods simply as input methods.
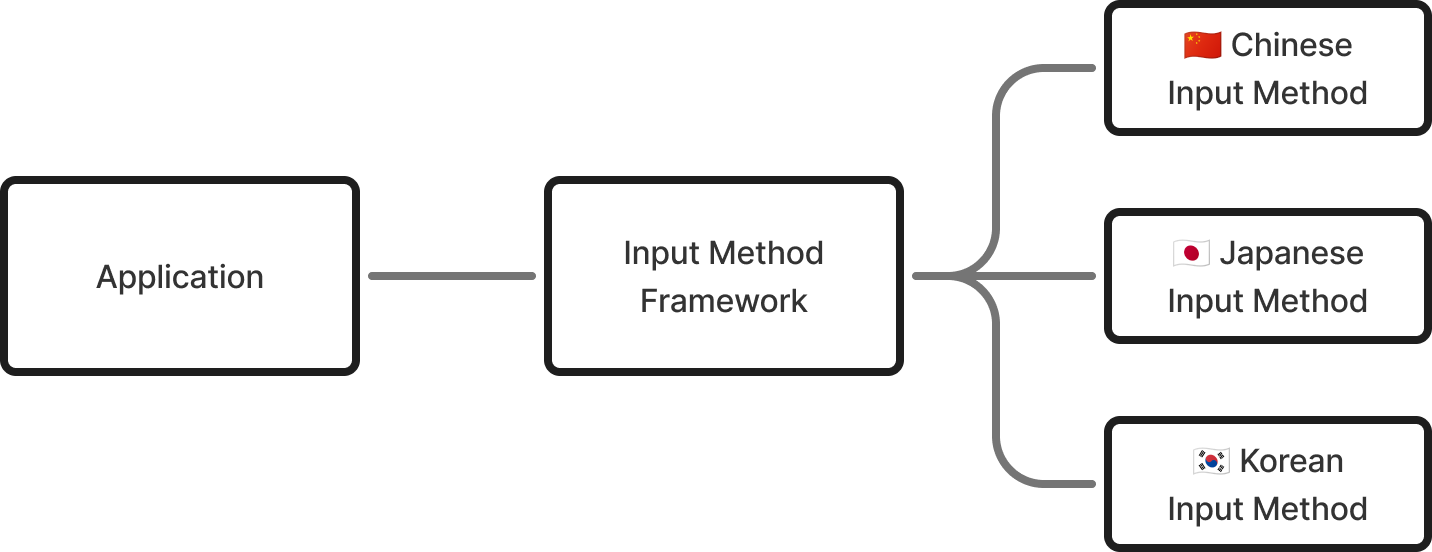
Input Method and Input Method Framework
2. Communication Protocols and Applications
Input methods are fundamentally designed with a client-server architecture. Applications can process inputs by communicating with the input method daemon through various IPC (Inter-Process Communication) methods. The protocols used in this process can be divided into two types: standard protocols that operate independently of the input method, and proprietary protocols developed by input method developers. Applications choose one of these communication methods to operate.
Standard Protocols
The standard protocols used for communication between clients and input methods are as follows:
X Input Method (XIM)
XIM is a protocol established by the X Consortium in the 1990s, defining how input methods and clients communicate through the X11 server. Being over 30 years old, it’s not commonly used nowadays, but most input methods still support it for compatibility. Although it’s X11-based, XIM protocol can still be used in Wayland environments when using XWayland, the X11 compatibility layer.
The following environment variable, often set when installing an input method, specifies which input method to use with the XIM protocol:
export XMODIFIERS=@im=ibus
Wayland
Wayland, a display protocol designed to replace X11, also includes protocols for input methods. Wayland’s input method protocol has changed twice, and even the latest version, text-input-unstable-v3, still has “unstable” in its name. However, at this point, text-input-unstable-v3 is effectively being used as the standard input protocol for Wayland. Interestingly, while XIM simply allows applications and input methods to communicate through the X11 server, text-input-unstable-v3 involves the Wayland compositor directly in the structure.
Own Protocols
Most input methods, such as IBus, Fcitx, and Uim, implement their own communication protocols in addition to standard protocols. IBus and Fcitx’s own protocols communicate over D-Bus, a Linux IPC system, while Uim uses sockets directly. These proprietary protocols and clients are primarily used for IM modules, which will be discussed later.
This raises the question of why own protocols were created despite the existence of standard protocols. We can speculate that the following process occurred:
- XIM, developed in the 1990s, only supported basic input functions and didn’t support modern input methods (such as surrounding text reference)
- Each input method development team (IBus, Fcitx, Uim, etc.) developed their own protocols to overcome XIM’s limitations
- Later, Wayland emerged, and three input protocols were created
- As a result, multiple protocols proliferated, and application developers had to support various protocols
Developing Applications That Use Input Methods
Application developers rarely need to consider input method-related issues.
In most cases, widget toolkits like GTK or Qt automatically handle integration with input methods behind the scenes.
So when using input fields like GtkEntry
or QLineEdit
in a widget toolkit, developers don’t need to worry about the details of input methods.
Widget toolkits typically support standard protocols like XIM or text-input-unstable-v3 natively, and code implementing an input method’s own protocol is usually loaded as a module/plugin. These are called IM modules. If you’ve installed an input method before, you’ve likely set environment variables like these to specify which IM module the widget toolkit should use:
export GTK_IM_MODULE=fcitx
export QT_IM_MODULE=fcitx
Applications with Custom UI Implementation
Not all applications use input fields provided by widget toolkits. Applications that implement and render their own UI, like web browsers, either implement standard input method protocols directly or use interfaces/classes provided by widget toolkits, such as Gtk.IMContext or QInputMethod, which abstract input methods to handle input for their custom UI elements.
Summary
The communication protocols of input methods and application structure described above can be visualized as follows:
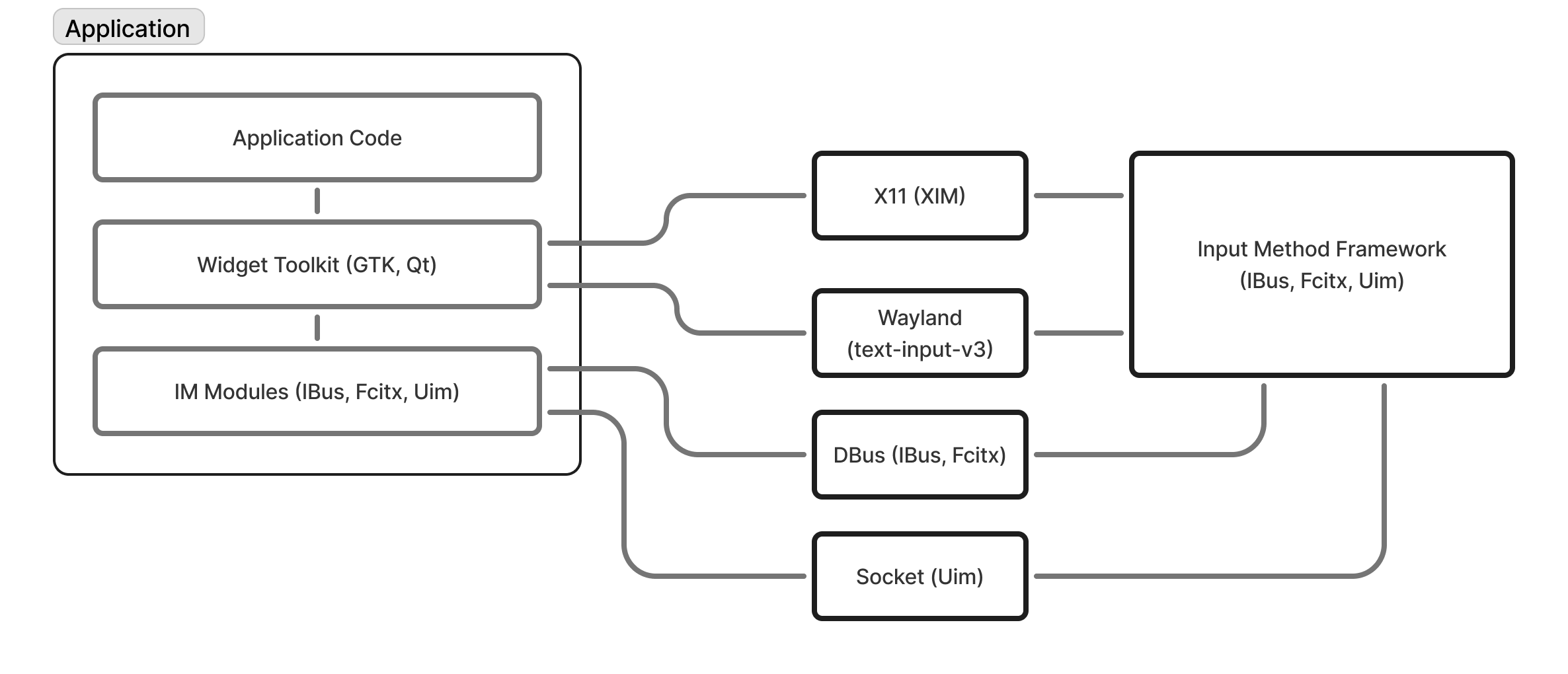
Input Method System Communication Structure Overview
3. General Operation Process of Input Methods
Let’s examine how input methods operate from key input to screen output from a programming perspective. This process is typically performed by widget toolkits like GTK or Qt.
To aid understanding, I’ve used JavaScript-based pseudocode.
Context Creation
A Context represents the state of a text input (text being composed, cursor position, etc.). Input methods use Contexts to maintain the input state of multiple input fields and applications as separate objects.
// Pseudocode
const inputContext = new InputContext();
Setting Cursor Position
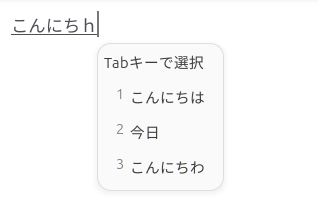
Input Candidate List
When inputting Japanese or Chinese, or using the Hanja key in Korean, input text candidates are displayed as shown above. This UI is displayed by the input method, and the application needs to send the screen coordinates of the current input cursor so that the input method can display it in the appropriate position. This also needs to be updated whenever the position of the input field changes, such as when the window moves.
// Pseudocode
inputContext.setCursorLocation(x, y, width, height);
Sending Key Input
Since input methods generally don’t receive key inputs directly from the display server, applications need to send key input events to the input method.2
The input method receives the key input and returns whether it processed the key. Keys that don’t require composition typically return false
.
For example, in a Korean input method, keys like numbers and symbols that can be input directly without composition fall into this category.
In such cases, applications typically process the original key as is.
// Pseudocode
function onKeyPress(keyCode) {
const isKeyProcessed = inputContext.processKey(keyCode);
// ...
}
Preedit Events
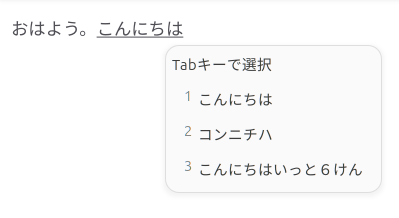
Preedit Text Example
When typing text, you may notice an underline appearing under characters that are still being composed. This text, which is still being input but not yet confirmed, is called Preedit text. When Preedit text is updated, the input method sends an event to the application, allowing users to see and edit text that hasn’t been composed yet in real-time.
// Pseudocode
function onPreeditTextUpdate(preeditText) {
// ...
}
// Add an event listener
inputContext.setOnPreeditTextUpdate(onPreeditTextUpdate);
Commit Events
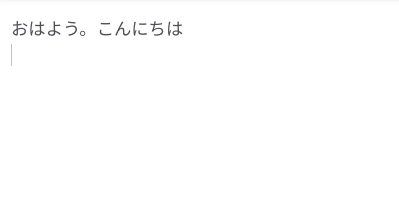
Commit Example
When a user finalizes the Preedit text they were inputting and sends it to the application, this is called a commit. Committed text is no longer in the special state managed by the input method, and the underline disappears. The conditions under which commits occur vary depending on the characteristics of the language being input and the input method being used, but generally, they occur when the Enter key is pressed, when a window is clicked with the mouse, or when the cursor is moved.
// Pseudocode
function onCommit(commitText) {
// ...
}
// Add an event listener
inputContext.setOnCommit(onCommit);
Try It Yourself
You can directly observe Preedit updates and Commits through the website below:
References
- IM module related
- IBus client and IM module implementation: https://github.com/ibus/ibus/tree/main/client
- Fcitx5 GTK IM: https://github.com/fcitx/fcitx5-gtk
- Fcitx5 QT IM: https://github.com/fcitx/fcitx5-qt
- Browser implementation of input methods in Linux environments using
Gtk.IMContext
- Flow of Japanese input in XIM: https://qiita.com/ai56go/items/63abe54f2504ecc940cd
-
Common GNU/Linux distributions using X11, Wayland, Freedesktop, etc. ↩︎
-
However, Wayland’s text-input-unstable-v3 protocol uses a method where the compositor sends key inputs to the input method and only sends the composition results to the application, so application key input transmission is not necessary. ↩︎